Automating Cisco Router Interaction with Python and Paramiko
Introduction: In the world of networking, managing Cisco routers efficiently is crucial for smooth operations. Automating routine tasks not only saves time but also reduces the risk of human errors. In this post, we’ll explore a Python script that leverages the Paramiko library to automate the login process to a Cisco router and retrieve interface information.
Script Overview: The provided Python script establishes an SSH connection to a Cisco router, logs in, enters privileged mode, and then executes the ‘show ip interface brief’ command to retrieve interface information.
Explanation of the Script:
-
- Importing Required Libraries:
import paramiko import time
The script begins by importing the Paramiko library for SSH communication and the time module for handling sleep functionality.
- Defining the Cisco Login Function:
def cisco_login(hostname, username, password, enable_password):
The
cisco_login
function is defined, which takes the Cisco router’s hostname, username, password, and enable password as parameters. - SSH Connection and Login Handling:
ssh = paramiko.SSHClient() ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
The script creates an SSH client and sets the policy for automatically adding the server’s host key.
- Establishing Connection and Shell Channel:
ssh.connect(hostname, username=username, password=password, allow_agent=False, look_for_keys=False) channel = ssh.invoke_shell()
The script connects to the router using provided credentials and creates a shell channel for interaction.
- Entering Privileged Mode:
channel.send("enable\n") channel.send(enable_password + "\n")
The script sends the ‘enable’ command and the enable password to enter privileged mode.
- Checking Successful Login:
enable_output = channel.recv(65535).decode('utf-8') if '#' in enable_output:
It checks if the prompt changes to ‘#’ in enable mode, indicating a successful login.
- Executing ‘show ip interface brief’ Command:
channel.send("show ip interface brief\n") ip_interface_brief_output = channel.recv(65535).decode('utf-8')
If in privileged mode, the script sends the ‘show ip interface brief’ command and retrieves the output.
- Closing the SSH Connection:
ssh.close()
The script closes the SSH connection after completing the tasks.
- Exception Handling:
except Exception as e: print(f"Error: {e}") print("Login failed!")
The script includes exception handling to print error messages in case of any issues during execution.
- Entry Point of the Script:
if __name__ == "__main__":
Conclusion: This Python script provides a foundation for automating Cisco router interactions, making it easier to retrieve critical information quickly and efficiently. This kind of automation can be extended to include additional commands and functionalities, offering a scalable solution for network management tasks.
Finally, the script defines the entry point, where you can replace the placeholder values with your actual router’s information and call the
cisco_login
function.
- Importing Required Libraries:
Here is the YouTube video:
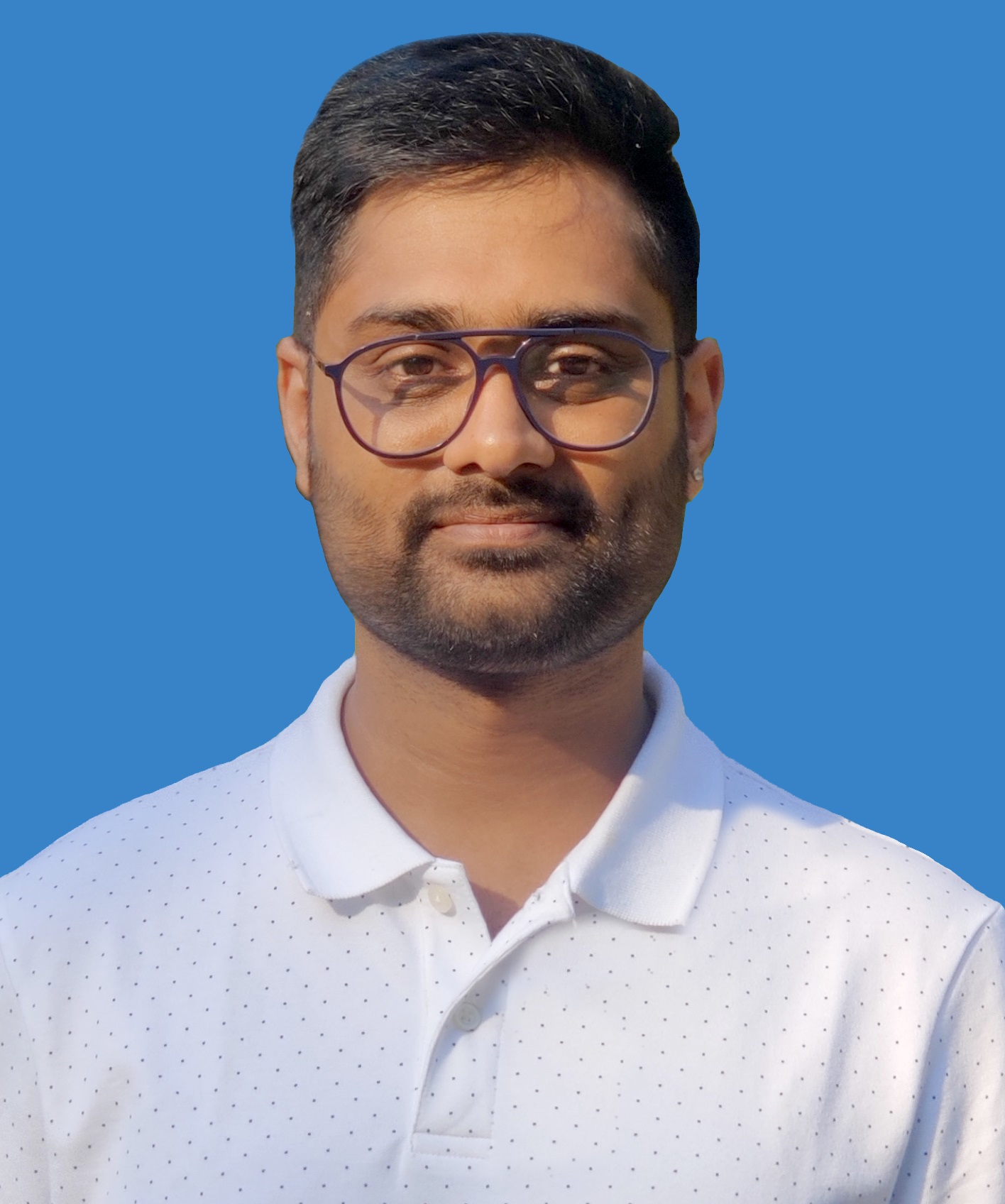
I am working in an IT company and having 10+ years of experience into Cisco IP Telephony and Contact Center. I have worked on products like CUCM, CUC, UCCX, CME/CUE, IM&P, Voice Gateways, VG224, Gatekeepers, Attendant Console, Expressway, Mediasense, Asterisk, Microsoft Teams, Zoom etc. I am not an expert but i keep exploring whenever and wherever i can and share whatever i know. You can visit my LinkedIn profile by clicking on the icon below.
“Everyone you will ever meet knows something you don’t.” ― Bill Nye