Automating Cisco Router Configuration with Python and Paramiko
Automating Cisco Router Configuration with Python and Paramiko
Introduction:
In the dynamic landscape of network management, automating routine tasks can significantly enhance efficiency and reduce the likelihood of errors. This post introduces a Python script powered by the Paramiko library, designed to automate the login and configuration process for Cisco routers. We’ll explore how this script establishes an SSH connection, logs in, enters privileged mode, and configures a loopback interface—all with just a few lines of code.
Script Overview:
# Import the Paramiko library for SSH communication import paramiko # Import the time module for sleep functionality import time # Define a function for Cisco login def cisco_login(hostname, username, password, enable_password): # Create an SSH client ssh = paramiko.SSHClient() # Automatically add the server's host key (this is insecure, see comments below) ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy()) try: # Connect to the router using provided credentials ssh.connect(hostname, username=username, password=password, allow_agent=False, look_for_keys=False) # Create a shell channel for interaction channel = ssh.invoke_shell() # Wait for the prompt after connecting time.sleep(1) # Receive and decode the initial output output = channel.recv(65535).decode('utf-8') print(output) # Check if login was successful by looking for '#' or '>' if '#' in output or '>' in output: # Send the 'enable' command to enter privileged mode channel.send("enable\n") time.sleep(1) # Send the enable password channel.send(enable_password + "\n") time.sleep(1) # Check if the prompt indicates successful enable mode enable_output = channel.recv(65535).decode('utf-8') print(enable_output) # Check if '#' is present in enable mode prompt if '#' in enable_output: print("Login successful! Entered privilege mode.") # Show initial IP interface brief channel.send("show ip interface brief\n") time.sleep(1) ip_interface_brief_output = channel.recv(65535).decode('utf-8') print(ip_interface_brief_output) # Configure loopback interface channel.send("conf t\n") time.sleep(1) channel.send("int loopback 0\n") time.sleep(1) channel.send("ip address 2.2.2.2 255.255.255.0\n") time.sleep(1) channel.send("end\n") time.sleep(1) # Show IP interface brief after configuration channel.send("show ip interface brief\n") time.sleep(1) ip_interface_brief_output = channel.recv(65535).decode('utf-8') print(ip_interface_brief_output) else: print("Enable mode failed!") print("Login failed!") else: print("Login failed!") # Close the SSH connection ssh.close() except Exception as e: # Handle exceptions and print an error message print(f"Error: {e}") print("Login failed!") # Entry point of the script if __name__ == "__main__": # Replace these values with your router's information router_hostname = "172.16.0.200" router_username = "cisco" router_password = "cisco@123" router_enable_password = "cisco@123" # Call the function to attempt login cisco_login(router_hostname, router_username, router_password, router_enable_password)
Explanation:
- SSH Connection and Login: The script establishes an SSH connection to the Cisco router, logs in, and enters privileged mode.
- Show Initial IP Interface Brief: It shows the initial ‘show ip interface brief’ output to provide a snapshot of the router’s interfaces.
- Configure Loopback Interface: The script enters configuration mode, creates a loopback interface, assigns an IP address, and ends the configuration.
- Show IP Interface Brief After Configuration: Finally, it displays the ‘show ip interface brief’ output again, showcasing the changes made to the router’s interfaces.
Conclusion: Automating Cisco router configurations with Python and Paramiko simplifies network management tasks. This script serves as a foundation, demonstrating how automation can streamline the login and configuration process, saving time and reducing the risk of errors in a network environment.
Here is the YouTube video:
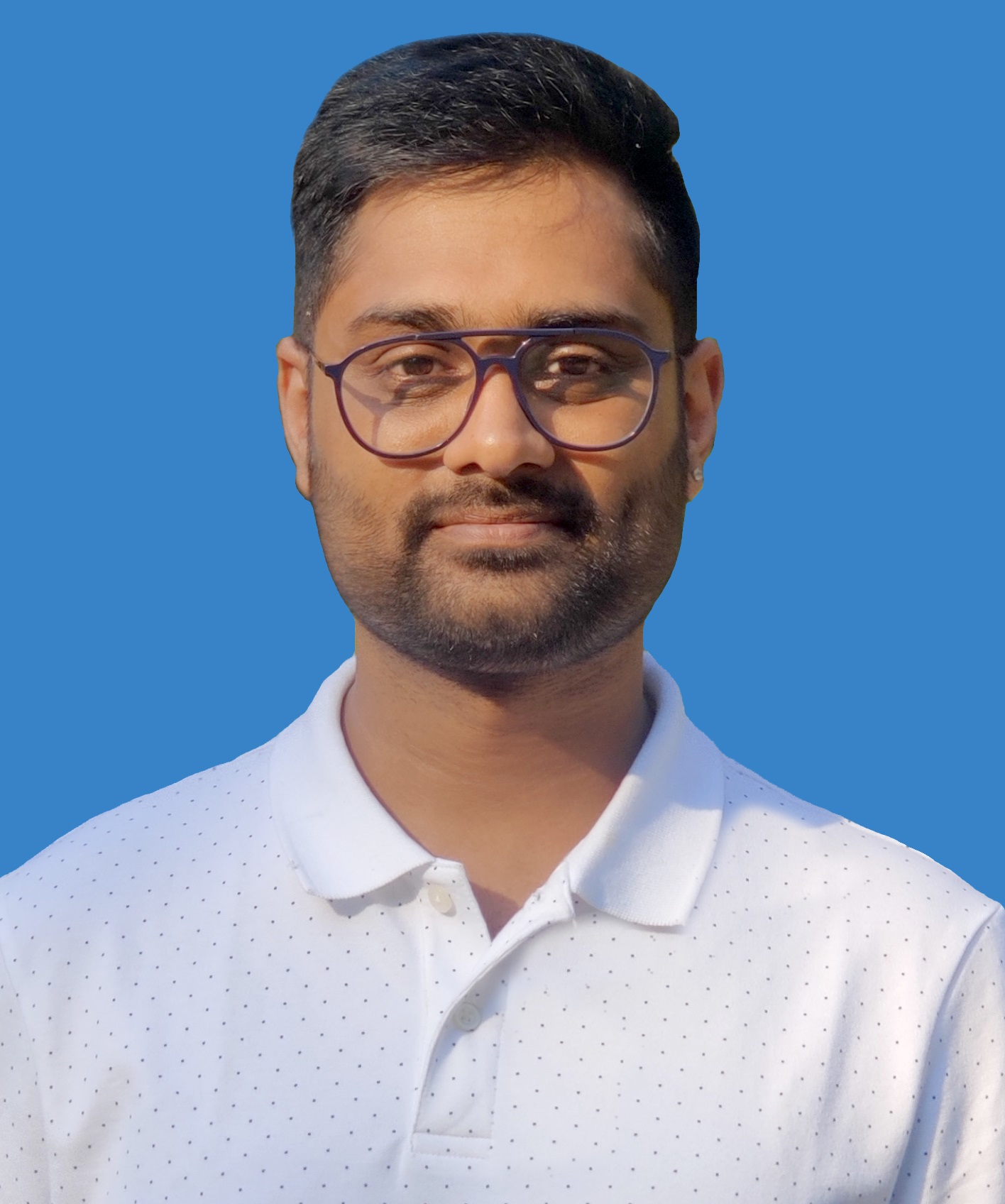
I am working in an IT company and having 10+ years of experience into Cisco IP Telephony and Contact Center. I have worked on products like CUCM, CUC, UCCX, CME/CUE, IM&P, Voice Gateways, VG224, Gatekeepers, Attendant Console, Expressway, Mediasense, Asterisk, Microsoft Teams, Zoom etc. I am not an expert but i keep exploring whenever and wherever i can and share whatever i know. You can visit my LinkedIn profile by clicking on the icon below.
“Everyone you will ever meet knows something you don’t.” ― Bill Nye