Automating Multiple Cisco Router Configuration Backup to SFTP Server with Python
Introduction:
In today’s dynamic networking landscape, the management and protection of device configurations play a pivotal role in ensuring network reliability and security. However, the manual retrieval and backup of configurations across multiple Cisco devices can be a tedious and error-prone task. This article explores a Python script powered by the Paramiko library, designed to automate the process of retrieving and securely transferring configurations from multiple Cisco routers and switches to a central repository via SFTP.
Automating Configuration Retrieval Across Multiple Devices:
The heart of this automation lies in the Python script, which intelligently connects to each Cisco device using SSH, navigates to privileged mode, and retrieves the running configuration. By leveraging the Paramiko library’s capabilities, this script streamlines the process of interacting with multiple devices, eliminating the need for manual intervention.
Secure Transfer to SFTP Server:
Once the configurations are fetched, the script seamlessly transfers them to a designated SFTP server. This ensures that the configurations are stored in a centralized and secure location, safeguarding against data loss and unauthorized access. With Paramiko’s robust SFTP functionality, the transfer process is encrypted and authenticated, adhering to stringent security standards.
Efficiency and Reliability at Scale:
One of the script’s most significant advantages is its ability to scale effortlessly across a fleet of Cisco devices. Whether managing a handful of routers or an extensive network infrastructure, the automation provided by Python and Paramiko remains consistent and reliable. Network administrators can execute the script with confidence, knowing that configurations are consistently and accurately backed up.
Enhancing Network Management Practices:
By automating the backup and transfer of Cisco configurations, network administrators can allocate their time and resources more efficiently. Tasks that once required manual attention can now be scheduled and executed seamlessly, freeing up valuable resources for more strategic initiatives. Moreover, with configurations securely stored on an SFTP server, organizations can mitigate the risk of configuration drift and ensure rapid recovery in the event of a network outage.
Conclusion:
In the ever-evolving landscape of network management, automation emerges as a cornerstone for efficiency and reliability. With Python and Paramiko, the process of retrieving and transferring Cisco configurations becomes a streamlined and scalable endeavor. By embracing automation, organizations can elevate their network management practices, fortifying their infrastructure against potential threats and disruptions.
Here is the script:
import paramiko import time def get_cisco_config(hostname, username, password, enable_password): try: # Connect to the Cisco device using SSH ssh_client = paramiko.SSHClient() ssh_client.set_missing_host_key_policy(paramiko.AutoAddPolicy()) ssh_client.connect(hostname, username=username, password=password) # Create a shell session shell = ssh_client.invoke_shell() # Send enable command shell.send("enable\n") time.sleep(1) shell.send(enable_password + "\n") # Send command to retrieve running configuration shell.send("ter len 0\n") # Setting up terminal length as 0 shell.send("show running-config\n") # Wait for the command to execute time.sleep(1) # Read the output of the command output = "" while shell.recv_ready(): output += shell.recv(1024).decode() # Close SSH connection ssh_client.close() return output except Exception as e: print(f"Error retrieving configuration from {hostname}: {e}") return None def upload_to_sftp(server, username, password, local_path, remote_path): try: # Establish an SFTP connection to the server transport = paramiko.Transport((server, 22)) transport.connect(username=username, password=password) sftp = paramiko.SFTPClient.from_transport(transport) # Upload the file sftp.put(local_path, remote_path) # Close SFTP connection sftp.close() print(f"Configuration uploaded to {server} at {remote_path}") except Exception as e: print(f"Error uploading configuration to {server}: {e}") if __name__ == "__main__": # List of Cisco device SSH credentials routers = [ {"hostname": "172.16.0.200", "username": "cisco", "password": "cisco", "enable_password": "cisco"}, {"hostname": "172.16.0.201", "username": "cisco", "password": "cisco", "enable_password": "cisco@123"}, # Add more routers as needed ] # SFTP server credentials sftp_server = "172.16.0.101" # IP address of the SFTP server sftp_username = "cisco" # Username for accessing the SFTP server sftp_password = "cisco" # Password for accessing the SFTP server for router in routers: config = get_cisco_config(router["hostname"], router["username"], router["password"], router["enable_password"]) if config: # Save running configuration to a local file local_path = f"running_config_{router['hostname']}.txt" with open(local_path, "w") as f: f.write(config) # Upload the running configuration to SFTP server upload_to_sftp(sftp_server, sftp_username, sftp_password, local_path, f"/running_config_{router['hostname']}.txt")
Here is the YouTube Video:
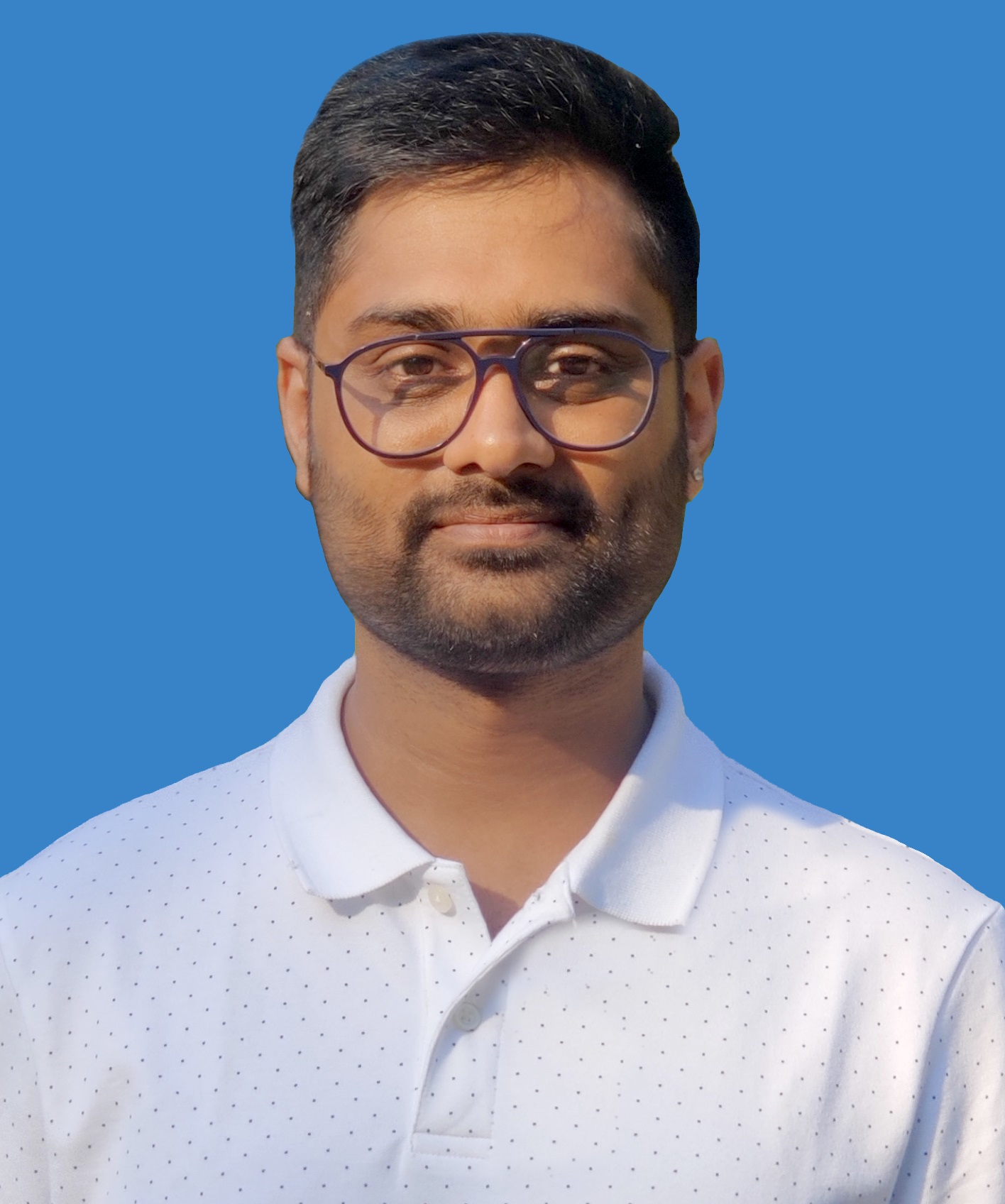
I am working in an IT company and having 10+ years of experience into Cisco IP Telephony and Contact Center. I have worked on products like CUCM, CUC, UCCX, CME/CUE, IM&P, Voice Gateways, VG224, Gatekeepers, Attendant Console, Expressway, Mediasense, Asterisk, Microsoft Teams, Zoom etc. I am not an expert but i keep exploring whenever and wherever i can and share whatever i know. You can visit my LinkedIn profile by clicking on the icon below.
“Everyone you will ever meet knows something you don’t.” ― Bill Nye