Automating Multiple Cisco Router and Switches Configuration Backup with Python Paramiko Reading CSV File
Automating Multiple Cisco Router and Switches Configuration Backup with Python Paramiko Reading CSV File
Managing network infrastructure, especially in large-scale environments, can be a daunting task. Manually backing up configurations from multiple Cisco routers and switches and then transferring them to a centralized repository is time-consuming and error-prone. However, with the power of Python and Paramiko, these tasks can be automated efficiently.
Introduction to the Script
Our Python script utilizes the Paramiko library, which provides SSH and SFTP capabilities, to automate the backup and transfer of configurations from Cisco devices to an SFTP server. Let’s dive into how the script works and how it can simplify network management processes.
Retrieving Configurations
The script begins by reading router information from a CSV file, which contains details such as the hostname, username, password, and enable password for each device. It then iterates through each router, establishing an SSH connection and sending commands to retrieve the running configuration. This is achieved by invoking a shell session on the device, sending the necessary commands, and capturing the output.
Uploading to SFTP Server
Once the running configuration is retrieved, the script saves it to a local file named after the router’s hostname. It then establishes an SFTP connection to the designated server using the provided credentials and uploads the configuration file to a specified directory on the server.
Benefits of Automation
Automating the backup and transfer of configurations offers several benefits:
- Time Savings: By eliminating manual processes, network administrators can save valuable time that can be allocated to more critical tasks.
- Consistency: Automation ensures that configurations are backed up consistently and accurately across all devices, reducing the risk of errors and inconsistencies.
- Security: Storing configurations in a centralized repository enhances security and facilitates auditing and compliance efforts.
- Scalability: The script can easily scale to manage configurations across a large number of devices, making it ideal for enterprise environments.
Conclusion
Automating configuration backup and transfer is essential for efficient network management. With our Python script leveraging Paramiko, network administrators can streamline these tasks, improve reliability, and free up time for more strategic initiatives. Embrace automation and take your network management practices to the next level.
Here is the link to the script:
import csv import paramiko import time def get_cisco_config(hostname, username, password, enable_password): try: # Connect to the Cisco device using SSH ssh_client = paramiko.SSHClient() ssh_client.set_missing_host_key_policy(paramiko.AutoAddPolicy()) ssh_client.connect(hostname, username=username, password=password) # Create a shell session shell = ssh_client.invoke_shell() # Send enable command shell.send("enable\n") time.sleep(1) shell.send(enable_password + "\n") # Send command to retrieve running configuration shell.send("ter len 0\n") # Setting up terminal length as 0 shell.send("show running-config\n") # Wait for the command to execute time.sleep(1) # Read the output of the command output = "" while shell.recv_ready(): output += shell.recv(1024).decode() # Close SSH connection ssh_client.close() return output except Exception as e: print(f"Error retrieving configuration from {hostname}: {e}") return None def upload_to_sftp(server, username, password, local_path, remote_path): try: # Establish an SFTP connection to the server transport = paramiko.Transport((server, 22)) transport.connect(username=username, password=password) sftp = paramiko.SFTPClient.from_transport(transport) # Upload the file sftp.put(local_path, remote_path) # Close SFTP connection sftp.close() print(f"Configuration uploaded to {server} at {remote_path}") except Exception as e: print(f"Error uploading configuration to {server}: {e}") if __name__ == "__main__": # Read router information from CSV file routers = [] with open('router_info.csv', 'r') as csvfile: csv_reader = csv.reader(csvfile) next(csv_reader) # Skip the header row for row in csv_reader: routers.append({ "hostname": row[0], "username": row[1], "password": row[2], "enable_password": row[3] }) # SFTP server credentials sftp_server = "172.16.0.101" # IP address of the SFTP server sftp_username = "cisco" # Username for accessing the SFTP server sftp_password = "cisco" # Password for accessing the SFTP server for router in routers: config = get_cisco_config(router["hostname"], router["username"], router["password"], router["enable_password"]) if config: # Save running configuration to a local file local_path = f"running_config_{router['hostname']}.txt" with open(local_path, "w") as f: f.write(config) # Upload the running configuration to SFTP server upload_to_sftp(sftp_server, sftp_username, sftp_password, local_path, f"/running_config_{router['hostname']}.txt")
Here is a sample CSV file:
hostname,username,password,enable_password 172.16.0.200,cisco,cisco,cisco 172.16.0.201,cisco,cisco,cisco@123
Here is the YouTube videos:
Stay tuned for more tutorials and insights into network automation and Python scripting!
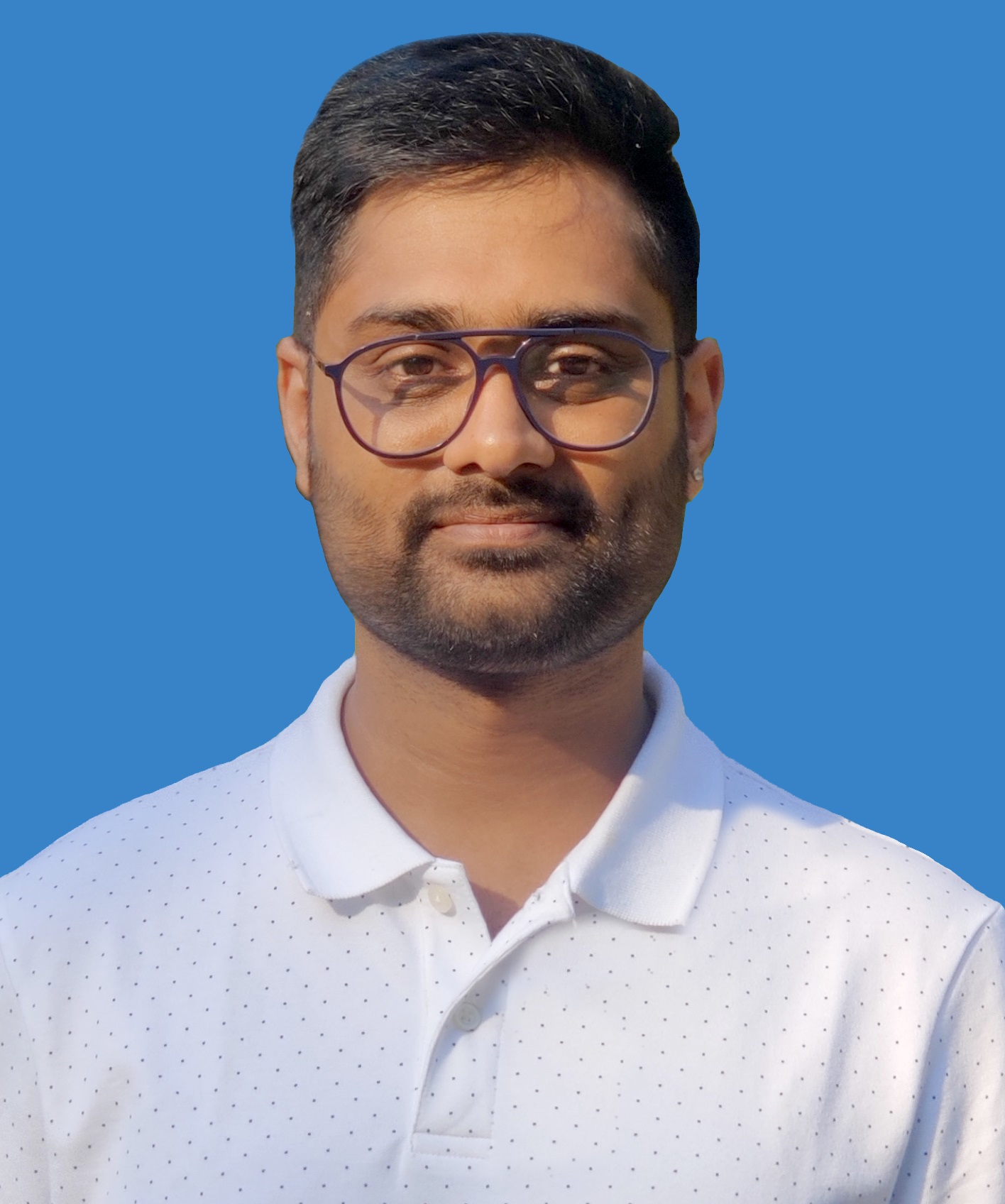
I am working in an IT company and having 10+ years of experience into Cisco IP Telephony and Contact Center. I have worked on products like CUCM, CUC, UCCX, CME/CUE, IM&P, Voice Gateways, VG224, Gatekeepers, Attendant Console, Expressway, Mediasense, Asterisk, Microsoft Teams, Zoom etc. I am not an expert but i keep exploring whenever and wherever i can and share whatever i know. You can visit my LinkedIn profile by clicking on the icon below.
“Everyone you will ever meet knows something you don’t.” ― Bill Nye