Automating Cisco Router Configuration Backup to SFTP Server with Python
Introduction: In the world of network management, ensuring the safety and accessibility of device configurations is paramount. Cisco devices, being the backbone of many networks, require robust backup mechanisms to prevent loss of critical configurations. Manual backups are tedious and error-prone, which is why automation comes to the rescue. In this article, we’ll explore how to automate the process of retrieving the running configuration from a Cisco device and transferring it to an SFTP server using Python and Paramiko.
- Importing Paramiko and Time Modules:
import paramiko import time
These lines import the necessary modules for SSH communication (
paramiko
) and time-related functions (time
). - Defining the
get_cisco_config
Function:def get_cisco_config(hostname, username, password, enable_password):
This line defines a function named
get_cisco_config
which takes four parameters:hostname
,username
,password
, andenable_password
. These parameters represent the credentials required to connect to the Cisco device. - Establishing SSH Connection to Cisco Device:
ssh_client = paramiko.SSHClient() ssh_client.set_missing_host_key_policy(paramiko.AutoAddPolicy()) ssh_client.connect(hostname, username=username, password=password)
Here, an SSH client object is created using Paramiko (
paramiko.SSHClient()
). Theset_missing_host_key_policy
method is called to automatically add the host key to the known hosts file. Then, theconnect
method is used to establish an SSH connection to the Cisco device using the provided credentials. - Invoking an Interactive Shell Session:
shell = ssh_client.invoke_shell()
This line invokes an interactive shell session on the SSH connection, allowing the execution of multiple commands and receiving their outputs.
- Sending Commands to the Cisco Device:
shell.send("enable\n") shell.send(enable_password + "\n") shell.send("ter len 0\n") shell.send("show running-config\n")
These lines send a series of commands to the Cisco device. First, it sends the
enable
command followed by the enable password to enter privileged mode. Then, it adjusts the terminal length to0
to ensure that the entire configuration is displayed without pagination. Finally, it sends theshow running-config
command to retrieve the running configuration. - Reading Output from the Shell:
output = "" while shell.recv_ready(): output += shell.recv(1024).decode()
Here, the script reads the output from the shell in a loop until there is no more data available (
shell.recv_ready()
). It concatenates the received data to theoutput
variable, which will contain the complete running configuration. - Closing the SSH Connection:
ssh_client.close()
Finally, the SSH connection to the Cisco device is closed to release resources and terminate the connection.
Benefits:
- Efficiency: Automation reduces manual effort and minimizes the risk of human error associated with manual backups.
- Reliability: Automated backups ensure that critical configurations are regularly saved and accessible when needed.
- Flexibility: Python’s versatility allows for customization and integration with other tools and processes in the network environment.
Conclusion: Automating Cisco configuration backup and transfer is a crucial aspect of network management, ensuring the safety and availability of critical configurations. By leveraging Python and Paramiko, network administrators can streamline this process, saving time and mitigating risks associated with manual operations. With automation, ensuring the integrity of Cisco configurations becomes a seamless and reliable task in the network management arsenal.
These key lines of code work together to establish an SSH connection to a Cisco device, retrieve its running configuration, and store it for further processing or backup purposes.
Here is the full configuration:
import paramiko # Importing the Paramiko library for SSH and SFTP functionality import time # Importing the time module for sleep function def get_cisco_config(hostname, username, password, enable_password): # Connect to the Cisco device using SSH ssh_client = paramiko.SSHClient() # Creating an SSH client object ssh_client.set_missing_host_key_policy(paramiko.AutoAddPolicy()) # Setting a policy for automatically adding host keys ssh_client.connect(hostname, username=username, password=password) # Establishing SSH connection to the Cisco device # Create a shell session shell = ssh_client.invoke_shell() # Invoking an interactive shell session for sending commands # Send enable command shell.send("enable\n") # Sending an 'enable' command to enter privileged mode time.sleep(1) # Waiting for a brief moment to ensure command execution shell.send(enable_password + "\n") # Sending the enable password to enter privileged mode # Send command to retrieve running configuration shell.send("ter len 0\n") # Setting up terminal length as 0 shell.send("show running-config\n") # Sending a command to retrieve the running configuration # Wait for the command to execute time.sleep(1) # Waiting for a brief moment to allow the command to execute # Read the output of the command output = "" # Initializing an empty string to store the command output while shell.recv_ready(): # Looping until data is available for receiving output += shell.recv(1024).decode() # Reading data from the shell and appending it to the output string # Close SSH connection ssh_client.close() # Closing the SSH connection return output # Returning the retrieved running configuration def upload_to_sftp(server, username, password, local_path, remote_path): # Establish an SFTP connection to the server transport = paramiko.Transport((server, 22)) # Creating a transport object for SFTP connection transport.connect(username=username, password=password) # Connecting to the SFTP server sftp = paramiko.SFTPClient.from_transport(transport) # Creating an SFTP client object from the transport # Upload the file sftp.put(local_path, remote_path) # Uploading the local file to the remote location on the SFTP server # Close SFTP connection sftp.close() # Closing the SFTP connection if __name__ == "__main__": # Cisco device SSH credentials cisco_hostname = "172.16.0.200" # IP address of the Cisco device cisco_username = "cisco" # SSH username for the Cisco device cisco_password = "cisco@123" # SSH password for the Cisco device cisco_enable_password = "cisco@123" # Enable password for the Cisco device (privileged mode) # SFTP server credentials sftp_server = "172.16.0.101" # IP address of the SFTP server sftp_username = "cisco" # Username for accessing the SFTP server sftp_password = "cisco1" # Password for accessing the SFTP server # Get Cisco running configuration config = get_cisco_config(cisco_hostname, cisco_username, cisco_password, cisco_enable_password) # Retrieving running configuration from the Cisco device # Save running configuration to a local file local_path = "running_config.txt" # Local file path to save the running configuration with open(local_path, "w") as f: # Opening the local file in write mode f.write(config) # Writing the running configuration to the local file # Upload the running configuration to SFTP server upload_to_sftp(sftp_server, sftp_username, sftp_password, local_path, "/running_config.txt") # Uploading the running configuration to the SFTP server
Here is the YouTube Video:
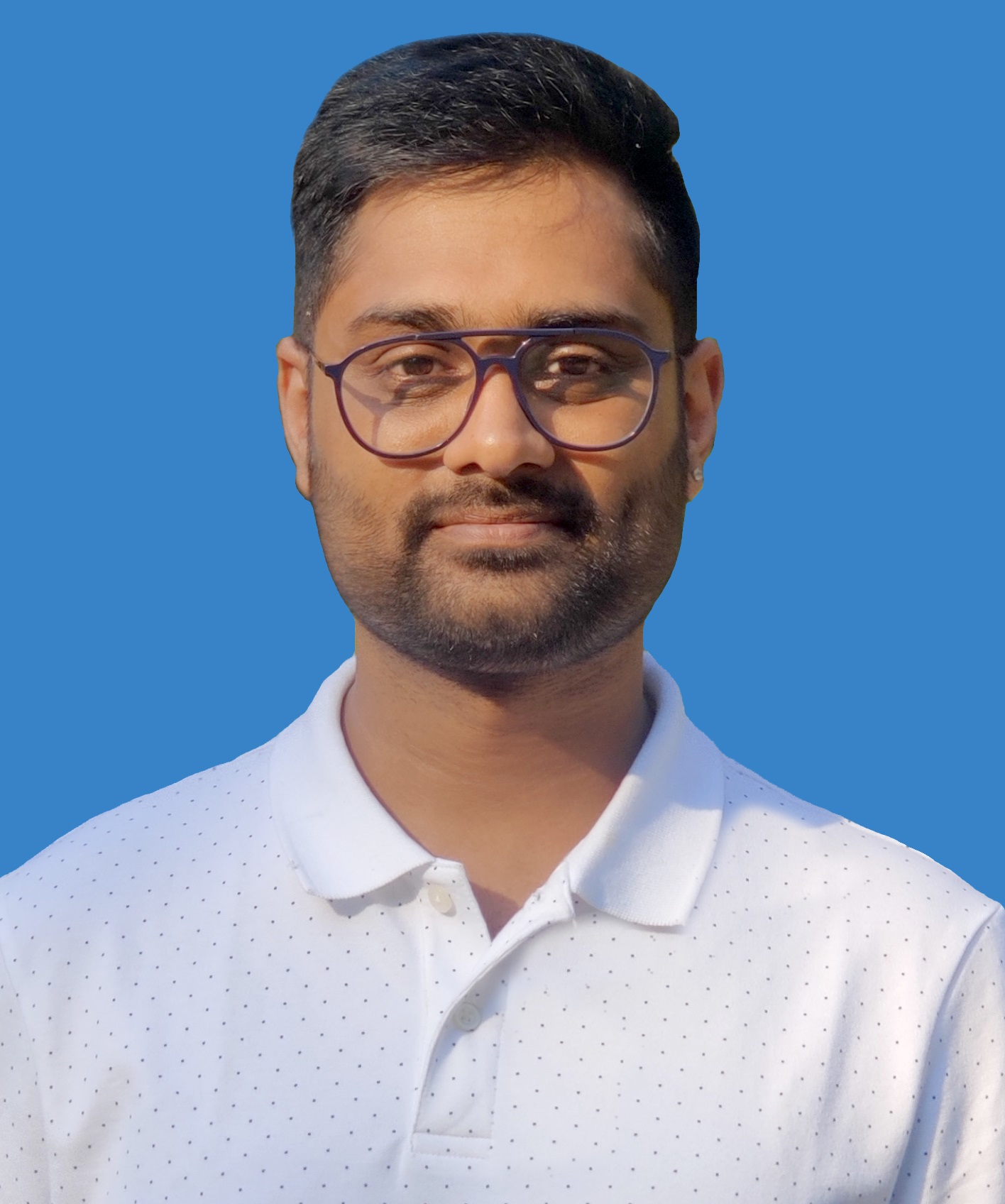
I am working in an IT company and having 10+ years of experience into Cisco IP Telephony and Contact Center. I have worked on products like CUCM, CUC, UCCX, CME/CUE, IM&P, Voice Gateways, VG224, Gatekeepers, Attendant Console, Expressway, Mediasense, Asterisk, Microsoft Teams, Zoom etc. I am not an expert but i keep exploring whenever and wherever i can and share whatever i know. You can visit my LinkedIn profile by clicking on the icon below.
“Everyone you will ever meet knows something you don’t.” ― Bill Nye