Secure Cisco Access: Simplifying SSH Login Automation with Python Paramiko
Secure Cisco Access: Simplifying SSH Login Automation with Paramiko
Introduction:
In the realm of network automation, secure access to Cisco devices is paramount. In this post, we explore a Python script that leverages the Paramiko library to automate SSH login to Cisco routers. This script not only streamlines the login process but also demonstrates how to enter privileged mode, providing network administrators with efficient and secure remote access.
Script Breakdown:
The script begins by importing the Paramiko library for SSH communication and the time module for sleep functionality. It defines a function, cisco_login
, which encapsulates the logic for SSH login to a Cisco router.
# Import the Paramiko library for SSH communication import paramiko # Import the time module for sleep functionality import time # Define a function for Cisco login def cisco_login(hostname, username, password, enable_password): # Create an SSH client ssh = paramiko.SSHClient() # Automatically add the server's host key (this is insecure, see comments below) ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy()) try: # Connect to the router using provided credentials ssh.connect(hostname, username=username, password=password, allow_agent=False, look_for_keys=False) # Create a shell channel for interaction channel = ssh.invoke_shell() # Wait for the prompt after connecting time.sleep(1) # Receive and decode the initial output output = channel.recv(65535).decode('utf-8') print(output) # Check if login was successful by looking for '#' or '>' if '#' in output or '>' in output: # Send the 'enable' command to enter privileged mode channel.send("enable\n") time.sleep(1) # Send the enable password channel.send(enable_password + "\n") time.sleep(1) # Check if the prompt indicates successful enable mode enable_output = channel.recv(65535).decode('utf-8') print(enable_output) # Check if '#' is present in enable mode prompt if '#' in enable_output: print("Login successful! Entered privilege mode.") else: print("Enable mode failed!") print("Login failed!") else: print("Login failed!") # Close the SSH connection ssh.close() except Exception as e: # Handle exceptions and print an error message print(f"Error: {e}") print("Login failed!") # Entry point of the script if __name__ == "__main__": # Replace these values with your router's information router_hostname = "172.16.0.200" router_username = "cisco" router_password = "cisco@123" router_enable_password = "cisco@123" # Call the function to attempt login cisco_login(router_hostname, router_username, router_password, router_enable_password)
The script then replaces the traditional Telnet approach with the more secure SSH protocol for communication with the Cisco router. It uses the Paramiko library to establish a secure connection, entering privileged mode by providing the enable password.
Conclusion:
This script exemplifies the importance of secure and automated SSH login to Cisco routers, showcasing the capabilities of the Paramiko library. Enhance your network automation toolkit by incorporating this script for efficient and secure remote access to Cisco devices. Stay tuned for more insights into network automation, as we continue to explore ways to optimize and streamline your networking experience.
Here is the link you YouTube video:
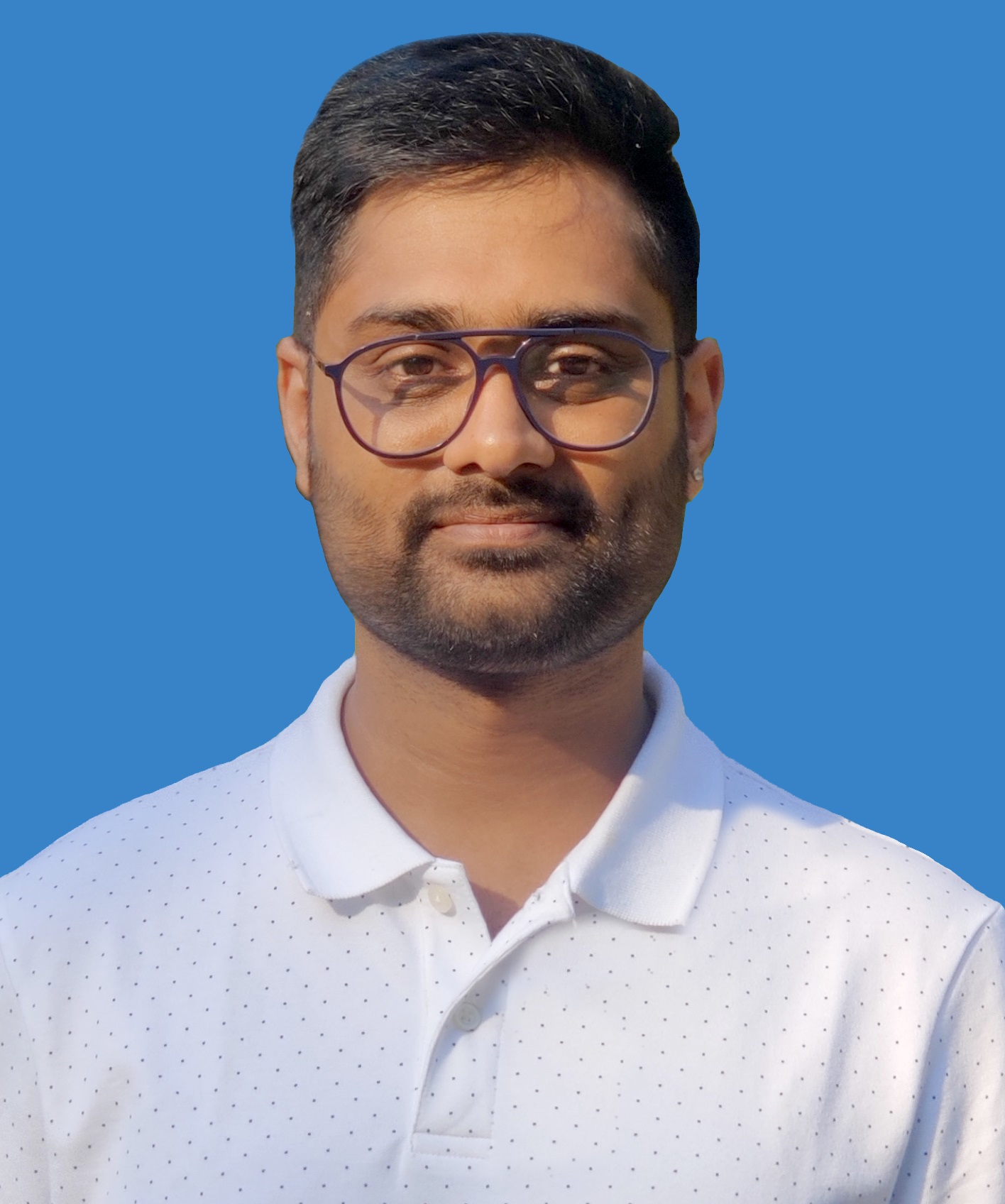
I am working in an IT company and having 10+ years of experience into Cisco IP Telephony and Contact Center. I have worked on products like CUCM, CUC, UCCX, CME/CUE, IM&P, Voice Gateways, VG224, Gatekeepers, Attendant Console, Expressway, Mediasense, Asterisk, Microsoft Teams, Zoom etc. I am not an expert but i keep exploring whenever and wherever i can and share whatever i know. You can visit my LinkedIn profile by clicking on the icon below.
“Everyone you will ever meet knows something you don’t.” ― Bill Nye