Python Automation on Cisco Router and Switches
Today in this article we will see how a python script automatically logs into a Cisco Router using telnet and configure a loopback interface.
Here is the working Python script:
import getpass import telnetlib HOST = "192.168.1.205" user = input("Enter your Username: ") password = getpass.getpass() enablepassword = getpass.getpass() tn = telnetlib.Telnet(HOST) tn.read_until(b"Username: ") tn.write(user.encode('ascii') + b"\n") if password: tn.read_until(b"Password: ") tn.write(password.encode('ascii') + b"\n") tn.read_until(b"R1>") tn.write(b"enable\n") tn.write(enablepassword.encode('ascii') + b"\n") tn.write(b"conf t\n") tn.write(b"int loop 0\n") tn.write(b"ip add 192.168.2.205 255.255.255.0\n") tn.write(b"no sh\n") tn.write(b"end\n") tn.read_until(b"R1#") tn.write(b"sh ip int brie\n") tn.write(b"exit\n") print(tn.read_all().decode('ascii'))
We are basically telling Python to:
- Telnet on 192.168.1.205
- Prompt for Username
- Prompt to type login password and also ensure that the typed characters are not displayed on the screen as we have used getpass module.
- As soon as we se R1> on the screen, we will execute “enable” command which should prompt to enter enable password.
- The python script then executes “conf t” and goes to global configuration mode
- Then it executes “int loop 0“
- Then it executes “ip add 192.168.2.205 255.255.255.0“
- Then it executes “no sh“
- Then it executes “end“
- Then it executes “sh ip int brie“
- Then it exits
- Then it prints the output.
Here is the output of the Python script execution:
PS C:\Users\Administrator\Desktop> python .\Telnet.py Enter your Username: cisco Password: Password: conf t Enter configuration commands, one per line. End with CNTL/Z. R1(config)#int loop 0 R1(config-if)#ip add 192.168.2.205 255.255.255.0 R1(config-if)#no sh R1(config-if)#end R1#sh ip int brie Interface IP-Address OK? Method Status Protocol Ethernet0/0 192.168.1.205 YES manual up up Ethernet0/1 unassigned YES unset administratively down down Ethernet0/2 unassigned YES unset administratively down down Ethernet0/3 unassigned YES unset administratively down down Loopback0 192.168.2.205 YES manual up up R1#exit PS C:\Users\Administrator\Desktop>
HTH.
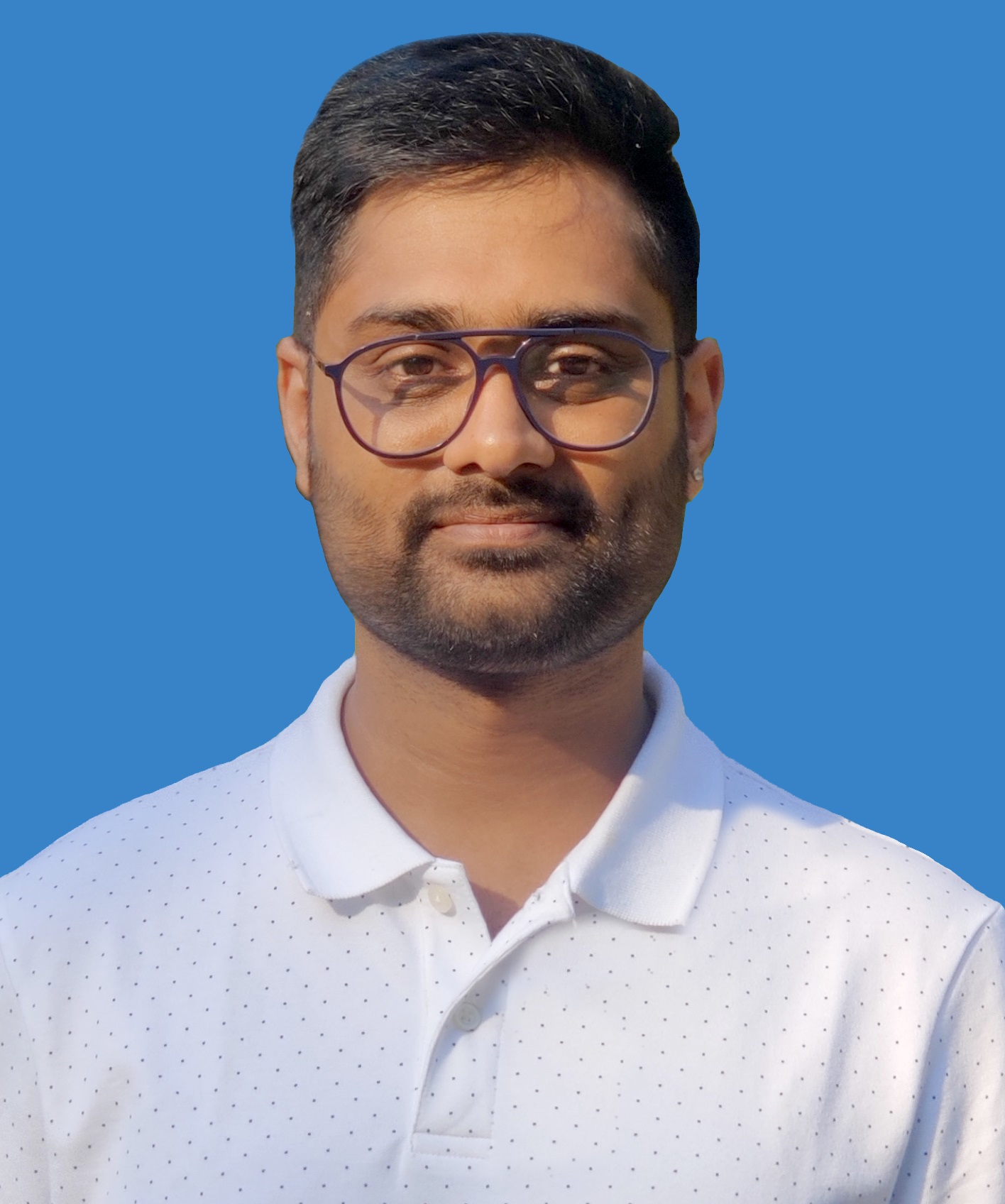
I am working in an IT company and having 10+ years of experience into Cisco IP Telephony and Contact Center. I have worked on products like CUCM, CUC, UCCX, CME/CUE, IM&P, Voice Gateways, VG224, Gatekeepers, Attendant Console, Expressway, Mediasense, Asterisk, Microsoft Teams, Zoom etc. I am not an expert but i keep exploring whenever and wherever i can and share whatever i know. You can visit my LinkedIn profile by clicking on the icon below.
“Everyone you will ever meet knows something you don’t.” ― Bill Nye
Thank you for sharing
This is a rather blunt way to do it. A more robust (But harder to setup way) is to use something like Expect.