Automating Common Router and Switches Configuration with Python, Paramiko and XML
In today’s fast-paced networking environments, automation plays a crucial role in efficiently managing network devices. In this post, we’ll explore how to automate router configuration using Python and Paramiko library, enabling you to streamline repetitive tasks and ensure consistency across your network infrastructure.
Introduction: Automating routine tasks such as router configuration can significantly enhance productivity and reduce the likelihood of human errors. Python, a versatile programming language, combined with Paramiko, a powerful SSH library, provides a robust solution for automating network device management.
Script Overview: The provided Python script demonstrates how to automate router configuration using SSH communication and XML-based configuration templates. Let’s break down its key components:
- Importing Libraries:
- The script imports essential libraries such as
csv
,paramiko
, andxml.etree.ElementTree
for handling CSV files, SSH communication, and XML parsing, respectively.
- The script imports essential libraries such as
- Function Definitions:
apply_router_config(hostname, username, password, enable_password, config_commands)
: This function establishes an SSH connection to the router specified by the provided hostname and applies configuration commands passed as arguments. It utilizes Paramiko for SSH communication and executes each command sequentially.main()
: The main function reads router credentials from a CSV file (router_credentials.csv
) and configuration commands from an XML file (router_configuration_template.xml
). It iterates over each router entry in the CSV file, applies the configuration commands, and prints success or error messages accordingly.
- Usage:
- To use the script, simply prepare a CSV file (
router_credentials.csv
) containing router credentials (hostname, username, password, enable password) and an XML file (router_configuration_template.xml
) containing the configuration template with commands to be applied.
- To use the script, simply prepare a CSV file (
- Example Workflow:
- Assume you have a network consisting of multiple routers that need to be configured with specific settings. By populating the CSV file with router credentials and XML file with configuration templates, you can execute the script to automate the configuration process for all routers in the network.
Conclusion: Automating router configuration using Python and Paramiko empowers network administrators to efficiently manage network devices, reduce manual errors, and ensure uniformity across the network infrastructure. By leveraging automation, you can streamline repetitive tasks and focus on strategic initiatives to drive business growth and innovation.
Here is the Python Script:
import csv # Import the CSV module for reading CSV files import paramiko # Import the Paramiko library for SSH communication import xml.etree.ElementTree as ET # Import the ElementTree module for parsing XML files import time # Import the time module for time-related functions def apply_router_config(hostname, username, password, enable_password, config_commands): try: # Create an SSH client object ssh_client = paramiko.SSHClient() # Set the policy for automatically adding host keys ssh_client.set_missing_host_key_policy(paramiko.AutoAddPolicy()) # Connect to the router using SSH ssh_client.connect(hostname, username=username, password=password) # Create a shell session for sending commands shell = ssh_client.invoke_shell() # Send the 'enable' command to enter privileged mode shell.send("enable\n") time.sleep(1) # Send the enable password shell.send(enable_password + "\n") # Send configuration commands one by one for cmd in config_commands: shell.send(cmd.strip() + "\n") # Strip leading/trailing spaces and send the command time.sleep(1) # Wait for a moment after sending each command # Save the configuration shell.send("end\n") time.sleep(1) shell.send("write memory\n") time.sleep(1) # Close the SSH connection ssh_client.close() # Print success message print(f"Configuration applied successfully for {hostname}") except Exception as e: # Print error message if configuration application fails print(f"Error applying configuration for {hostname}: {e}") def main(): # Read router information from the CSV file with open('router_credentials.csv', 'r') as csvfile: csv_reader = csv.reader(csvfile) # Create a CSV reader object next(csv_reader) # Skip the header row for row in csv_reader: # Iterate over each row in the CSV file hostname, username, password, enable_password = row # Extract router credentials from the row # Read configuration commands from the XML file tree = ET.parse('router_configuration_template.xml') # Parse the XML file root = tree.getroot() # Get the root element of the XML tree config_commands = [cmd.text for cmd in root.findall('config/command')] # Extract configuration commands # Apply the configuration to the router apply_router_config(hostname, username, password, enable_password, config_commands) if __name__ == "__main__": main() # Call the main function if the script is executed directly
Here is the CSV file: Filename – router_credentials.csv
hostname,username,password,enable_password 172.16.0.200,cisco,cisco,cisco 172.16.0.201,cisco,cisco,cisco@123
Here is the XML file: Filename – router_configuration_template.xml
<router_config> <config> <command>conf t</command> <command>ntp server 172.16.0.1</command> <command>clock timezone IST 5 30</command> <command>logging buffered 10000000</command> <command>access-list 1 permit 172.16.1.0 0.0.0.255</command> <command>access-list 1 deny any</command> <command>snmp-server community public RO</command> <command>ssh timeout 120</command> <command>no telnet-server</command> <command>int loop 0</command> <command>ip add 1.1.1.1 255.255.255.0</command> </config> </router_config>
Here is the YouTube Video:
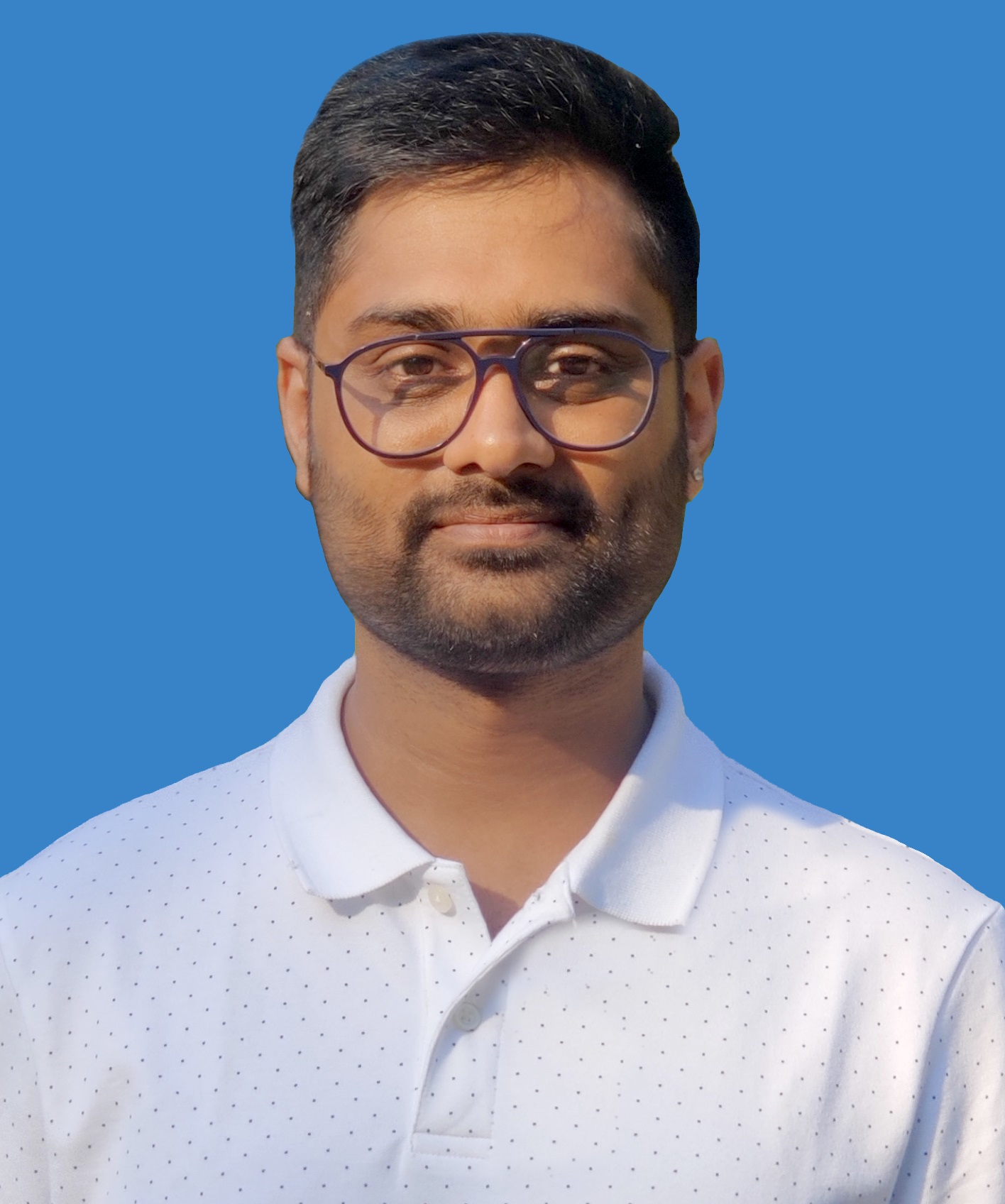
I am working in an IT company and having 10+ years of experience into Cisco IP Telephony and Contact Center. I have worked on products like CUCM, CUC, UCCX, CME/CUE, IM&P, Voice Gateways, VG224, Gatekeepers, Attendant Console, Expressway, Mediasense, Asterisk, Microsoft Teams, Zoom etc. I am not an expert but i keep exploring whenever and wherever i can and share whatever i know. You can visit my LinkedIn profile by clicking on the icon below.
“Everyone you will ever meet knows something you don’t.” ― Bill Nye